목표 |
객체를 생성 한 후에 객체가 계속 남아 있으면 메모리를 계속 차지하게 된다. 따라서 객체가 사망하거나 없어지는 시점에 객체를 소멸해 주어야 메모리에서 해제 되므로 이러한 객체 삭제 함수에 대해 알아 보자.
이론 |
Destroy() : 오브젝트 삭제 함수로 매개변수에 입력 되어 있는 오브젝트를 삭제 하는 함수
매개변수
object : 삭제할 게임 오브젝트
Time : 지연 시간
실습 |
시작 할때 객체를 생성 후에 3초 후에 삭제 되는 프로그램을 작성해 보자.
2022.05.30 - [응용프로그래밍/유니티기초] - [유니티기초]1-06.스크립트를 이용하여 객체 생성
에서 생성한 객체를 3초 후에 삭제 되도록 코딩 해 보자.
private void Awake() {
//Quaternion.identity 회전값(0,0,0)
Quaternion rotation = Quaternion.Euler(0,0,0); //각도를 나타내는 오일러를 Quaternion 으로 변환
GameObject c1=Instantiate(circle,new Vector3(0,3,0),rotation);
c1.name = "동그라미1";
c1.GetComponent<SpriteRenderer>().color = Color.yellow; //생성된 객체 색상 변경
c1.transform.position = new Vector3(-3,3,0); //생성된 객체 위치 변경
c1.transform.localScale = new Vector3(0.5f,0.5f,0); //생성된 객체 크기 변경
Destroy(c1,3f);
}
연습문제 |
1. 플레이어가 스페이스를 누르면 총알이 나가고 3초후에 총알이 사라지는 프로그램을 만들어 봅시다.
플레이어 코드
void Update()
{
if(Input.GetKeyDown(KeyCode.Space)){
Instantiate(circle,FirePos.transform.position,FirePos.transform.rotation);
}
}
FirePos 은 총알이 발사될 위치를 게임오브젝트로 생성
총알 생성 위치
Rigidbody2D rb;
public float speed = 500f;
// Start is called before the first frame update
void Start()
{
rb = GetComponent<Rigidbody2D>();
rb.AddForce(new Vector2(speed, 0));
Destroy(gameObject,3.0f);
}
2. 플레이어가 이동하면서 총을 쏘는 동작을 수행하자.
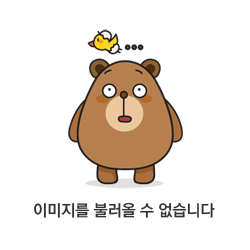
플레이어
void Update()
{
// 수평과 수직 축 입력 값을 감지하여 저장
float xInput = Input.GetAxis("Horizontal");
//float zInput = Input.GetAxis("Vertical");
float yInput = Input.GetAxis("Vertical");
// 실제 이동 속도를 입력 값과 이동 속력을 통해 결정
float xSpeed = xInput * speed;
//float zSpeed = zInput * speed;
float ySpeed = yInput * speed;
// Vector3 속도를 (xSpeed, ySpeed, zSpeed)으로 생성
Vector3 newVelocity = new Vector3(xSpeed, ySpeed, 0);
// 리지드바디의 속도에 newVelocity를 할당
rb.velocity = newVelocity;
if(Input.GetKeyDown(KeyCode.Space)){
Instantiate(circle,FirePos.transform.position,FirePos.transform.rotation);
}
}
3. 총알이 일정 범위를 벗어나면 총알이 사라지도록 처리하자.
이동 하는 위치
transform.positoin.x , transform.positoin.y 축이 일정 범위 밖으로 나가면 Destroy 해 주면 된다.
4. 벽을 생성해서 총알이 벽에 맞으면 총알을 삭제 하고 벽의 색깔을 잠깐 붉은 색으로 변하도록 처리해 보자.
1. 총알에 Collider 2D 설정
2. 벽을 세운 후 Box Collider 2D 를 추가 후 Is Trigger 체크 후 다음과 같이 스크립트 연결
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class wallScript : MonoBehaviour
{
private SpriteRenderer spriteRenderer;
// Start is called before the first frame update
private void Awake() {
spriteRenderer = GetComponent<SpriteRenderer>();
}
private void OnTriggerEnter2D(Collider2D other) {
//벽에 부딪힌 오브젝트를 삭제
Destroy(other.gameObject);
//충돌이 일어나면 벽의 색상을 바꿔 보자
StartCoroutine("Hit"); //코루틴을 이용하여 Hit 를 실행 시키자.
}
private IEnumerator Hit(){
spriteRenderer.color = Color.red;
yield return new WaitForSeconds(0.5f);
spriteRenderer.color = Color.white;
}
void Start()
{
}
// Update is called once per frame
void Update()
{
}
}
'응용프로그래밍 > 유니티기초' 카테고리의 다른 글
[유니티기초]1-10. 카메라 이동 (0) | 2022.05.30 |
---|---|
[유니티기초]1-09. 객체 방향 전환 (0) | 2022.05.30 |
[유니티기초]1-07. Random.Range 를 이용하여 랜덤한 위치에 객체 생성 (0) | 2022.05.30 |
[유니티기초]1-06.스크립트를 이용하여 객체 생성 (0) | 2022.05.30 |
[유니티기초]1-05. 프레임에 따라 update 되는 속도가 다른 것을 어느 컴퓨터에서나 동일한 속도를 보장 하는 deltaTime (0) | 2022.05.30 |