반응형
목표 |
플레이어를 앞과 뒤쪽을 바라 볼 수 있도록 방향 회전 하는 방법을 살펴 보자.
이론 |
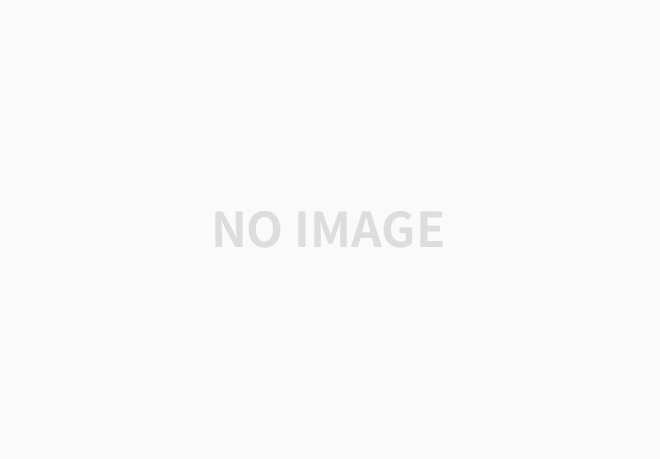
Rotation 이 y가 0 인 경우
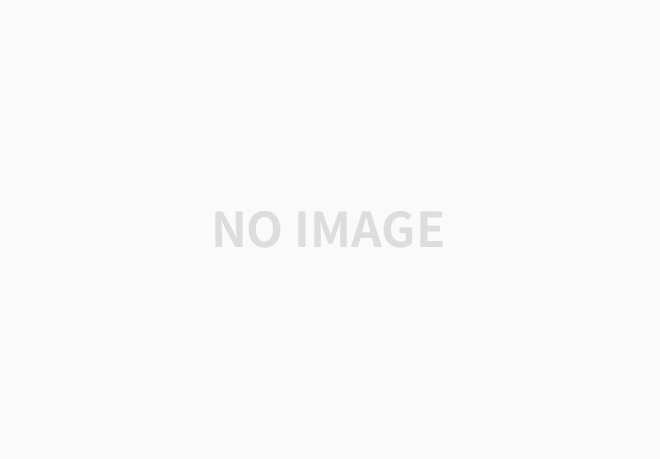
y가 180인경우
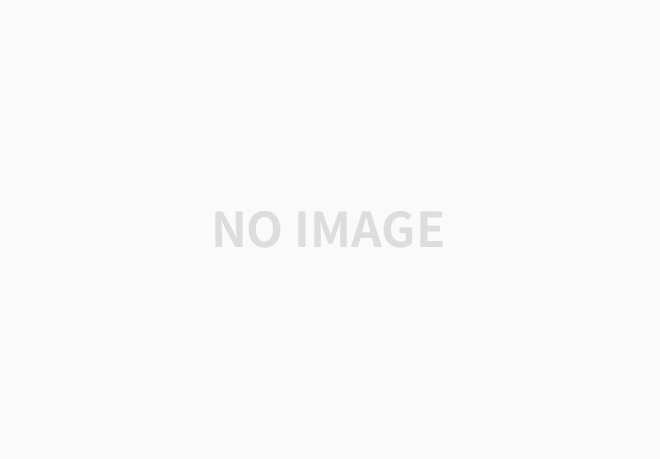
방향을 변경하는 것은
transform.rotation 값을 이용하여 변경한다.
이때 값은 Quaternion 값을 활용하는데 Quaternion.Euler를 이용하여 Euler(오일러)값을 변환하여 사용할 수 있다.
실습 |
왼쪽 방향키를 누르면 왼쪽을 바라보도록 처리하고
오른쪽 방향키를 누르면 오른쪽 방향을 바라보도록 처리하자.
void Update()
{
// 수평과 수직 축 입력 값을 감지하여 저장
float xInput = Input.GetAxis("Horizontal");
//float zInput = Input.GetAxis("Vertical");
float yInput = Input.GetAxis("Vertical");
if(xInput<0){
//방향 회전
transform.rotation = Quaternion.Euler(0,180,0);
}
else if(xInput>0){
transform.rotation = Quaternion.Euler(0,0,0);
}
}
오른쪽 방향을 바라보면 오른쪽으로 총알이 나가도록 수정해 보자.
총알의 스크립트를 다음과 같이 변경후에 생성하는 부분에서 방향을 설정하자.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CircleMove : MonoBehaviour
{
//Rigidbody2D rb;
public float speed = 1.0f;
private Vector3 moveDir;
// Start is called before the first frame update
void Start()
{
//rb = GetComponent<Rigidbody2D>();
//rb.AddForce(new Vector2(speed, 0));
Destroy(gameObject,3.0f);
}
public void Setup(Vector3 dir){
moveDir = dir;
//Debug.Log("Setup");
}
// Update is called once per frame
void Update()
{
//새로운 위치 = 현재 위치 + (방향 * 속도)
transform.position += moveDir * speed * Time.deltaTime;
}
}
생성하는 스크립트
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ObjectSpawner : MonoBehaviour
{
public GameObject circle;
public Transform FirePos;
// Start is called before the first frame update
Rigidbody2D rb; //Rigidbody 를 연결할 변수 선언
public float speed = 10f; // 이동 속도
Vector3 dir;
private void Awake() {
}
void Start()
{
rb = GetComponent<Rigidbody2D>(); //자신의 Rigidbody 를 가져와서 rb에 연결
dir = Vector3.right;
}
// Update is called once per frame
void Update()
{
// 수평과 수직 축 입력 값을 감지하여 저장
float xInput = Input.GetAxis("Horizontal");
float yInput = Input.GetAxis("Vertical");
if(xInput<0){
//방향 회전
transform.rotation = Quaternion.Euler(0,180,0);
dir = Vector3.left;
//Debug.Log("Left");
}
else if(xInput>0){
transform.rotation = Quaternion.Euler(0,0,0);
dir = Vector3.right;
//Debug.Log("Right");
}
// 실제 이동 속도를 입력 값과 이동 속력을 통해 결정
float xSpeed = xInput * speed;
float ySpeed = yInput * speed;
// Vector3 속도를 (xSpeed, ySpeed, zSpeed)으로 생성
Vector3 newVelocity = new Vector3(xSpeed, ySpeed, 0);
// 리지드바디의 속도에 newVelocity를 할당
rb.velocity = newVelocity;
if(Input.GetKeyDown(KeyCode.Space)){
GameObject clone = Instantiate(circle,FirePos.transform.position,FirePos.transform.rotation);
clone.GetComponent<CircleMove>().Setup(dir);
}
}
}
총알을 생성하면서 방향을 셋팅해 주면 총알은 방향을 따라서 진행하게 된다.
연습문제 |
1. 총알이 좌우뿐만 아니라 마지막으로 상하좌우 키를 받아서 해당 방향으로 움직일 수 있도록 구현해 보자.
더보기
if(xInput<0){
//방향 회전
transform.rotation = Quaternion.Euler(0,180,0);
dir = Vector3.left;
//Debug.Log("Left");
}
else if(xInput>0){
transform.rotation = Quaternion.Euler(0,0,0);
dir = Vector3.right;
//Debug.Log("Right");
}
if(yInput<0){
//방향 회전
dir = Vector3.down;
//Debug.Log("Left");
}
else if(yInput>0){
dir = Vector3.up;
}
반응형
'응용프로그래밍 > 유니티기초' 카테고리의 다른 글
[유니티기초]2-01.에셋스토어에서 2D 이미지 다운받아 적용하기 (0) | 2022.05.31 |
---|---|
[유니티기초]1-10. 카메라 이동 (0) | 2022.05.30 |
[유니티기초] 1-08.유니티 객체 삭제 (0) | 2022.05.30 |
[유니티기초]1-07. Random.Range 를 이용하여 랜덤한 위치에 객체 생성 (0) | 2022.05.30 |
[유니티기초]1-06.스크립트를 이용하여 객체 생성 (0) | 2022.05.30 |