https://getcodingkids.com/mission/mission-3/
Mission 3 - Get Coding!
In Mission 3 you are going to learn how to build an app using HTML and JavaScript. An app, which is a short for “application”, is a type of program built to help a user with a specific task. In this mission you are going to code a to-do list app. The u
getcodingkids.com
목표
- 자바 스크립트로 버튼 만들기
- DOM을 사용하여 HTML 다루기
- localStorage API를 이용해 웹페이지에 있는 정보 저장하기
- 할 일 목록 앱 만들기
자바스크립트로 버튼만들기
- input 태그를 사용해서 사용자가 클릭 할 수 있는 버튼을 만든다.
<!DOCTYPE html>
<html>
<head>
<title>To-Do List App</title>
<style>
input[type="button"] {
background-color: pink;
}
</style>
</head>
<body>
<p>Special Exhibition To-Do List</p>
<br/>
<input type="text" value="Type here to add task"/>
<br/>
<input type="button" value="Add item"/>
<br/>
</body>
</html>
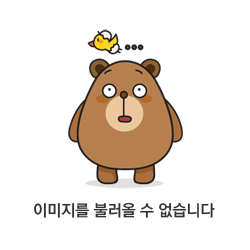
<input/> 태그의 type을 사용해 글상자와 버튼을 만들었다.
- 버튼 실행 코드 만들기
onClick 속성을 추가하여 클릭 할 때 자바스크립트가 실행 되도록 처리해 보자.
<!DOCTYPE html>
<html>
<head>
<title>To-Do List App</title>
<script>
function addItem() {
alert("Hire security team!");
}
</script>
</head>
<body>
<p>Special Exhibition To-Do List App</p>
<br/>
<input type="text" value="Type here to add task"/>
<br/>
<input type="button" value="Add item" onclick="addItem();"/>
<br/>
</body>
</html>
버튼을 클릭했을 때 다음과 같은 메시지가 출력 되는 것을 확인 할 수 있다.
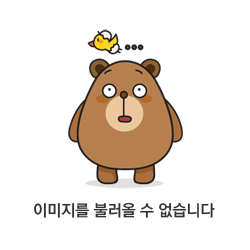
DOM을 사용하여 HTML 다루기
DOM을 사용하여 HTML 요소를 변경하는 방법을 살펴 보자.
DOM(Document Object Model)은 웹브라우저에서 동작하는 빌트인 함수를 모아놓은 것이다.
이러한 빌트인 함수를 모아 놓은 것을 API(Application Program Interface)라고 한다.
도큐먼트를 구성하는 HTML개체는 부모,자식 관계처럼 서로 연결되어 있다.
- HTML 요소의 내용 변경
<!DOCTYPE html>
<html>
<head>
<title>Methods</title>
<script>
function addItem() {
document.getElementById("container").innerHTML = "Item to remember";
}
</script>
</head>
<body>
<input type="button" value="Add item" onclick="addItem();"/>
<div id="container"></div>
</body>
</html>
getElementById 메소드는 id 속성을 사용하여 요소를 찾는데 사용된다.
innerHTML 속성을 사용하여 HTML 요소의 내용을 변경한다.
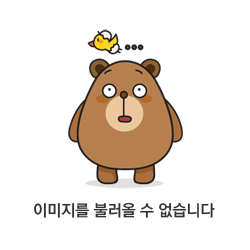
버튼 클릭시 container 요소의 값에 Item to remember 로 변경하는 것을 확인 할 수 있다.
- HTML에 새로운 요소 추가
createElement 및 AppendChild 메서드를 사용하여 앱에 새 HTML요소를 추가하는 방법을 살펴 보자
<!DOCTYPE html>
<html>
<head>
<title>New Elements</title>
<script>
function addItem() {
var newItem = document.createElement("div");
newItem.innerHTML = "New item";
document.getElementById("list").appendChild(newItem);
}
</script>
</head>
<body>
<div id="list" onclick="addItem();">Click here to add item</div>
</body>
</html>
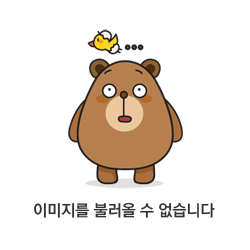
- HTML 요소 제거
RemoveChild 메서드를 사용하여 앱에서 HTML 요소를 제거하는 방법을 살펴 보자.
<!DOCTYPE html>
<html>
<head>
<title>Remove Items</title>
<script>
function removeItem(item) {
document.getElementById("list"). removeChild(item);
}
</script>
</head>
<body>
<div id="list">
Security to protect Mr Volkov
<div onclick="removeItem(this);">
Woolly socks
</div>
</div>
</body>
</html>
- 둘 이상의 HTML 요소 제거
<!DOCTYPE html>
<html>
<head>
<title>To-Do List App</title>
<script>
function addItem() {
var newItem = document.createElement("div");
newItem.innerHTML = document.getElementById("box").value;
newItem.onclick = removeItem;
document.getElementById("list").appendChild(newItem);
}
function removeItem() {
document.getElementById("list").removeChild(this);
}
</script>
</head>
<body>
<p>Special Exhibition To-Do List</p>
<br/>
<input type="text" id="box" value="Type here to add task"/>
<br/>
<input type="button" value="Add item" onclick="addItem();"/>
<br/>
<div id="list"></div>
</body>
</html>
자바스크립트의 onClick 속성에 removeItem 이 실행되면서 객체가 삭제 된다.
localStorage API 사용
새로고침을 눌러 웹페이지를 다시 불러오거나 웹브라우저를 닫았다 다시 실행하면 할 일 목록이 사라지는 것을 막기 위해 HTML에서 사용 가능한 localStorage을 사용해 정보를 저장하는 방법을 살펴 보자.
localStorage.storageName = "information"
여기서 storageName 은 저장할 정보의 이름이다.
만약 해당 정보를 지우고 싶다면
localStorage.storageName = ""
빈 값을 설정하여 삭제한다.
<!DOCTYPE html>
<html>
<head>
<title>Storage</title>
<script>
function save() {
var newItem = document.getElementById("box").value;
localStorage.box = newItem;
}
function load() {
var savedDiv = document.getElementById("savedList");
savedDiv.innerHTML = localStorage.box;
}
</script>
</head>
<body>
<input type="text" id="box" value="Type here to add task"/><br/>
<input type="button" id="save" value="Save" onclick="save();"/><br/>
<input type="button" id="load" value="Load" onclick="load();"/><br/>
Saved item: <div id="savedList"></div>
</body>
</html>
할일 목록 앱 구축하기
<!DOCTYPE html>
<html>
<head>
<title>To-Do List App</title>
<script>
function addItem() {
var newItem = document.createElement("div");
newItem.innerHTML = document.getElementById("box").value;
newItem.onclick = removeItem;
document.getElementById("list").appendChild(newItem);
saveList();
}
function removeItem() {
document.getElementById("list").removeChild(this);
saveList();
}
function saveList() {
localStorage.storedList = document.getElementById("list").innerHTML;
}
function loadList() {
document.getElementById("list").innerHTML = localStorage.storedList;
for(var i = 0; i < list.children.length; i++) {
list.children[i].onclick = removeItem;
}
}
</script>
<style>
.header {
height:75px;
width: 350px;
background-color:lightblue;
float: center;
padding:10px;
margin:20px;
text-align:centre;
font-size:24px;
}
.app {
height:600px;
width: 350px;
background-color:lightgreen;
float: center;
padding:10px;
margin:20px;
}
input[type="button"] {
background-color: pink;
}
</style>
</head>
<body>
<div class="header">
<img src="volkovlogo.png" style="float: left;">
<p>The House of Volkov</p>
</div>
<div class="app">
<p style="font-size:18px; font-style:bold;">Special Exhibition To-Do List</p>
<input type="text" id="box" value="Type here to add task"/>
<br/>
<input type="button" value="Add item" onclick="addItem();"/>
<br/>
<div id="list"></div>
</div>
<script>
if(localStorage.storedList) {
loadList();
}
</script>
</body>
</html>
addItem 을 호출하면 목록에 항목을 추가 후 saveList를 호출한다.
saveList 에서는 list 항목에 있는 내용을 저장한다.
loadList 에서는 localStorage에 있는 내용을 모두 불러와서 list 안에 추가한다음
list에 있는 자식들의 갯수만큼 모두 removeItem을 onClick 이벤트에 추가한다.
'웹프로그래밍 > HTML5+CSS3+JavaScript' 카테고리의 다른 글
[코딩 자율학습 HTML + CSS]2.1HTML의 기본 구성 요소 (0) | 2024.02.27 |
---|---|
[do it]프런트엔드UI개발 7. SPA 기본골격 만들기2 (0) | 2023.12.29 |
미션2.암호입력 웹페이지 제공 (1) | 2023.11.14 |
미션1. 웹페이지 구축 (1) | 2023.11.13 |
부트스트랩 강의 및 템플릿 사이트 (0) | 2023.11.06 |